목록React (13)
개발공부일지
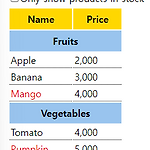
https://react.dev/learn/thinking-in-react - 메뉴판 만들기 (table) function ProductTable({ products, filterText, inStockOnly }) { const rows = []; let lastCategory = null; products.forEach((product) => { if (product.name.toLowerCase().indexOf(filterText.toLowerCase()) === -1) return; if (inStockOnly && !product.stocked) return; if (product.category !== lastCategory) { rows.push( ); } rows.push(); lastC..
목차 1. children props 2. Hooks ① useRef ② useMemo ③ useContext ④ useCallback ⑤ useReducer ⑥ custom hook : useInput 1. children props - 주로 컴포넌트 간의 데이터 전달이나 구조를 유연하게 관리하기 위해 사용 (어떤 내용이든 전달할수있음!) - 부모 컴포넌트는 자식 컴포넌트에게 데이터, 함수 또는 JSX 요소와 같은 내용을 전달할 수 있음 - 코드의 재사용성을 높이고 컴포넌트 간의 결합도를 낮출 수 있다고한다! export const LoginButton = ({ children }) => { return {children}; }; 2. Hooks ① useRef https://react.dev/refe..
목차 1. 요구사항 2. App에서 설정 3. 회원가입 4. 메인페이지 5. 글쓰기 6. 글보기 7. 글 수정하기 완성 코드 github → https://github.com/subinkr/react-pair-coding 1. 요구사항 - user 회원가입, 로그인, 마이페이지 - board 리스트(main), 글쓰기, 보기, 수정하기, 삭제하기 - 회원가입하고 로그인해야 글쓰기가 가능 - 수정, 삭제도 작성자일 경우 가능 2. App에서 설정 - Routes, Route, useState 사용 - [ user, setUser], [ userList, setUserList ], [ boardList, setBoardList ] 각 페이지에 맞는 상태를 props로 전달해주기 3. 회원가입 - userna..
목차 1. React Router Hook 2. Styled Component 3. 고차 컴포넌트(HOC, Higher Order Component) 4. Atomic Pattern 1. React Router Hook - 리액트의 페이지는 어떤 컴포넌트를 보여줄지 URL로 요청 보냈을때 - 페이지 전환 이벤틀르 막고 - URL 내용을 가지고 조건 처리해주면 컴포넌트가 바껴서 페이지 전환된것처럼 보임 - 쇼핑몰 페이지에서 - params 값을 가지고 처리한다거나, query 로 처리하는데 ---> hook을 사용 - 상품을 검색했을때 state 상태값에만 저장하면 - 검색한것을 브라우저가 url 저장해두면 공유한다면 /shop/params - URL 파라미터일 경우 : /:shop/:type/:optio..
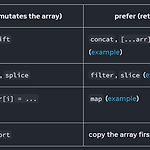
1. Updating Objects in State (마우스 포인터) https://react.dev/learn/updating-objects-in-state import { useState } from "react"; export default function MovingDot() { const [position, setPosition] = useState({ x: 0, y: 0, }); return ( { setPosition({ x: e.clientX, y: e.clientY, }); }} style={{ position: "relative", width: "100vw", height: "100vh", }} > ); } - 상태를 읽기전용으로 처리 - 새로운 객체를 만들어서 설정함수에 전달해서 다시..
https://react.dev/learn/queueing-a-series-of-state-updates 1. 요청 카운터 수정하기 - 카운터를 늘리거나 줄이는 클릭중에 결정된 구체적인 값("pending") 으로 설정하면 업데이트를 할수있다! async function handleClick() { setPending((p) => p + 1); await delay(3000); setPending((p) => p - 1); setCompleted((c) => c + 1); } - 클릭했을때 pending 상태값에 +1 (현재 pending 상태값에 이전값인 p를 이용해서 업데이트) - delay함수로 3초를 기다림 - 3초가 경과하면 setPending로 pending 상태값에서 -1 - setCompl..
https://react.dev/learn/reacting-to-input-with-state# Reacting to Input with State – React The library for web and native user interfaces react.dev 1. input 상태에 따라 시각적으로 상태 식별하기 - 비어있는지, 입력중인지, 제출하는지, 성공했는지, 오류인지를 시각적으로 나타내주 import { useState } from "react"; export default function Form() { const [answer, setAnswer] = useState(""); const [error, setError] = useState(null); const [status, setStat..
Passing Props to a Component https://react.dev/learn/passing-props-to-a-component Passing Props to a Component – React The library for web and native user interfaces react.dev props 전달하기!!! import { getImageUrl } from "./utils.js"; function Profile({ person, size = 80 }) { const imageSrc = getImageUrl(person); return ( {person.name} Profession: {person.profession} Awards: {person.awards.length} ..
목차 1. react router dom 2. BrowserRouter 3. Routes, Route 4. Link 1. react router dom - 설치하기 npm install react-router-dom@6 2. BrowserRouter // index.js import { BrowserRouter } from "react-router-dom"; const root = ReactDOM.createRoot(document.getElementById("root")); root.render( ); 3. Routes, Route - 위치가 변경될때마다 의 하위경로를 찾아 일치하는 항목을 랜더링해준다. // app.js import { Routes, Route } from "react-router-d..
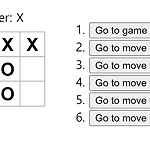
목차 1. 리액트 튜토리얼 따라하기 2. Lifting state up 3. immutability (불변성) 4. Winner 설정 5. Time Travel 6. Picking a Key 7. 완성코드 1. 리액트 튜토리얼 따라하기 https://react.dev/learn/tutorial-tic-tac-toe https://codesandbox.io/p/sandbox/exciting-rubin-5clxn7?file=%2FApp.js%3A1%2C1-151%2C1 2. Lifting state up export default function Board() { const [squares, setSquares] = useState(Array(9).fill(null)); function handleClick..